In object-oriented programming, the concept of IS-A is a totally based on Inheritance, which can be of two types Class Inheritance or Interface Inheritance. It is just like saying "A is a B type of thing". For example, Apple is a Fruit, Car is a Vehicle etc. Inheritance is uni-directional. For example, House is a Building. But Building is not a House.
#Is-A relationship --> By Inheritance
class A:
def __init__(self):
self.b=10
def mym1(self):
print('Parent method')
class B(A):
def mym2(self):
print('Child method')
d = B()
d.mym1()
#output: Parent method
d.mym2()
#output: Child method
HAS-A Relationship:
Composition(HAS-A) simply mean the use of instance variables that are references to other objects. For example Maruti has Engine, or House has Bathroom.
Let’s understand these concepts with an example of Car class.
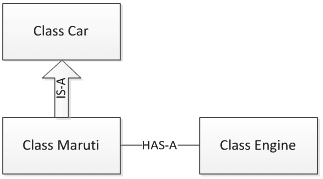
# Has-A relationship --> By Composition
class Engine:
def __init__(self):
self.b = 20
def myEngine(self):
print('This is Engine class')
class Car:
def __init__(self):
self.engine = Engine()
def mycar(self):
print('Car use Engine class functionality')
print(self.engine.b)
self.engine.myEngine()
a = Car()
a.mycar()
Output:
Car use Engine class functionality
20
This is Engine class
A program to show differentiation between Is-A and Has-A
class Car:
def __init__(self,name,colour):
self.name = name
self.colour = colour
def Printinfo(self):
print('car name is {},colour is {}'.format(self.name,self.colour))
class Person:
def __init__(self,name,age):
self.name = name
self.age = age
def breathe(self):
print('breathing')
class Employee(Person):
def __init__(self,name,age,empno,car):
super().__init__(name,age)
self.empno = empno
self.car = car #Has-A
def empinfo(self):
print('name is {},age is {},empno is {}'.format(self.name,self.age,self.empno)) #is-A
def empcarinfo(self):
print('car details:')
self.car.Printinfo()
c = Car('swift','red')
emp1 = Employee('tom',24,14356,c)
emp1.empinfo()
name is tom,age is 24,empno is 14356
emp1.empcarinfo()
car details:
car name is swift,colour is red
emp1.breathe()
breathing
Comments
Post a Comment